Welcome to a comprehensive guide on integrating Firebase Authentication with Spring Boot. This article will walk you through setting up and using Firebase to create secure REST APIs with Spring Security. Let’s dive in!
Why Use Firebase with Spring Boot?
Firebase offers a backendless approach to application development, enabling you to build powerful apps without the need for a dedicated backend. However, there are scenarios where communication with an existing API or a dedicated backend is necessary. That’s where this Spring Boot Starter comes into play, allowing you to expand Firebase’s authentication features alongside Spring Security for a seamless experience.
Configuration Steps
To get started, you need to set up some configurations:
- Make sure to configure the following environment variable: GOOGLE_APPLICATION_CREDENTIALS=path_to_firebase_server_config.json
- Decide whether the starter should use Firebase sessions on the client-side, server-side, or both.
- Be aware that HttpOnly Secure enabled session cookies may have compatibility issues while developing on localhost or 127.0.0.1. Implementing a self-signed SSL certificate can help resolve these challenges. You can explore this further in the article on Local Domain Names with SSL for Development Applications.
- Adjust the application properties to meet your specific needs, which you can find a sample of in application.yaml.
Understanding Role Management
Effective role management is crucial for controlling access to your APIs and UI components:
- Roles can be assigned during user registration or manually managed by super admins.
- Identify super admins through the application property security.super-admins.
- Utilize Spring Security annotations like @Secured, @RolesAllowed, and @PreAuthorize to enforce access controls.
- Define specific role annotations such as @IsSuper and @IsSeller for simplicity and clarity.
For example, the following code allows access to protected data for sellers:
@GetMapping(data)
@isSeller
public String getProtectedData() {
return "You have accessed seller only data from Spring Boot";
}
UI Integration
Utilize the useAuth hook to expose utility properties such as roles, hasRole, isSuper, and isSeller across your application, enabling or restricting UI component access as needed.
For a detailed explanation on role management, check out the post on Firebase and Spring Boot Based Role Management and Authorization.
End-to-End Testing with Firebase
Testing Firebase social authentication can be challenging. To address this, a toggleable Test User functionality can be created, authenticating a specific set of static test users via Firebase custom tokens. This approach solves many issues related to testing third-party authentication flows. More on this can be found in the post on End to End Test Firebase Authentication with Cypress, Spring Boot & Next.js.
Troubleshooting
If you encounter issues during setup or operation, here are some troubleshooting tips:
- Ensure the GOOGLE_APPLICATION_CREDENTIALS path is correctly set and accessible.
- Check your environment settings, especially if using self-signed SSL certificates.
- Verify role annotations are correctly defined to avoid access issues.
For more insights, updates, or to collaborate on AI development projects, stay connected with fxis.ai.
Final Thoughts
Integrating Firebase Authentication with Spring Boot can enhance your application’s security and functionality. Ensure you follow the configurations carefully to take full advantage of the robust features offered by Firebase and Spring Security.
At fxis.ai, we believe that such advancements are crucial for the future of AI, as they enable more comprehensive and effective solutions. Our team is continually exploring new methodologies to push the envelope in artificial intelligence, ensuring that our clients benefit from the latest technological innovations.
Related Tutorials
- Firebase Authentication for Spring Boot Rest API
- Firebase and Spring Boot Based Role Management and Authorization
- Firebase with Spring Boot for Kubernetes Deployment Configuration
- Local Domain Names with SSL for Development Applications
- Firebase Server Side Session Authentication with Next.js and Spring Boot
- End to End Test Firebase Authentication with Cypress, Spring Boot & Next.js
UI Demos
- Next.js Application Demonstrating Client Side Firebase Session
- Next.js Application Demonstrating Server Side Firebase Session
Screenshots
Client Side Session Screenshots
Logged out:
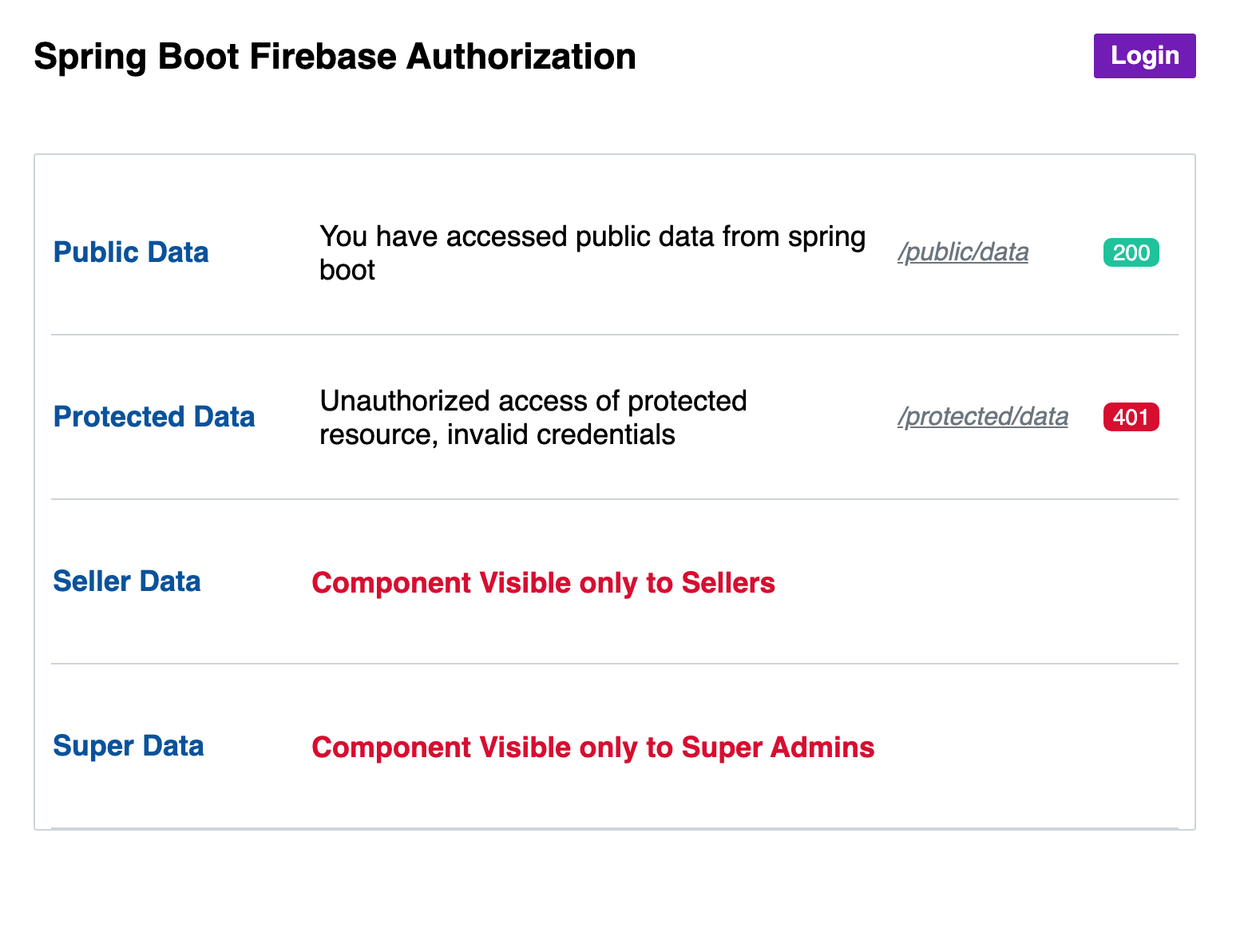
Logged In:
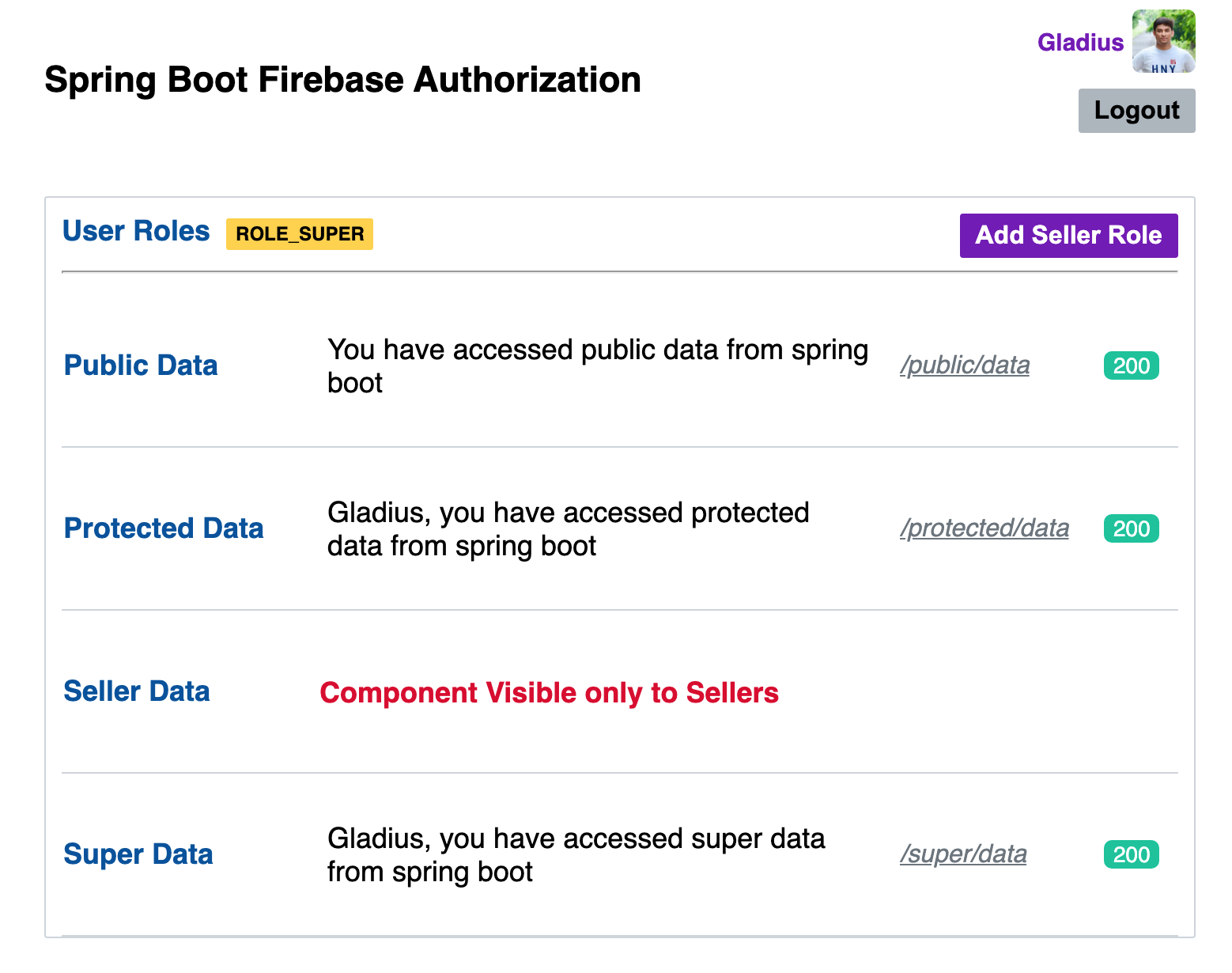
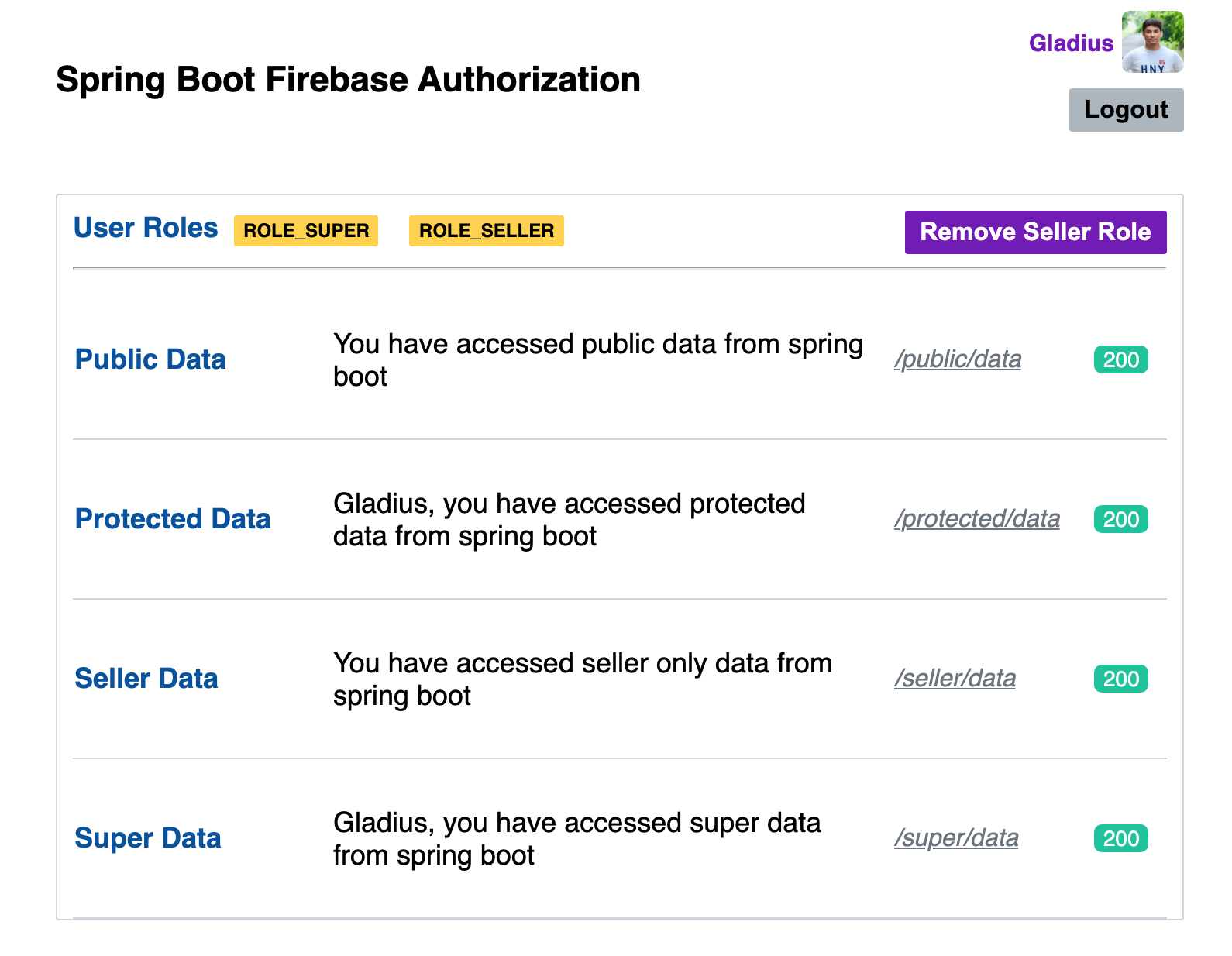
Server Side Session Screenshots
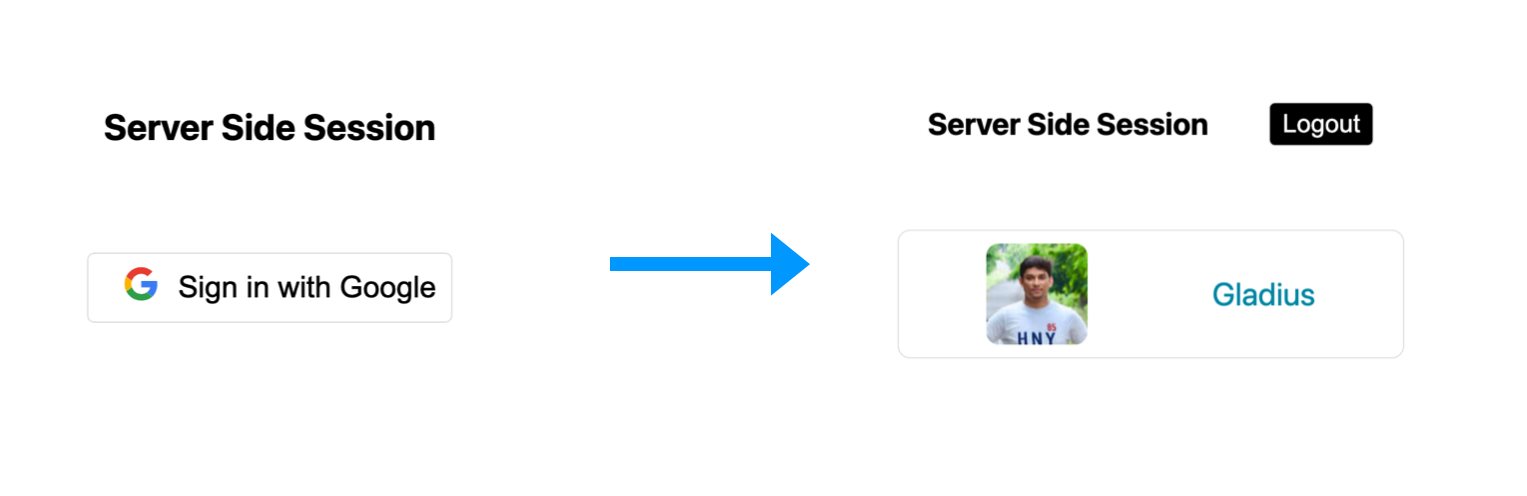
Cypress End to End Tests Screencapture
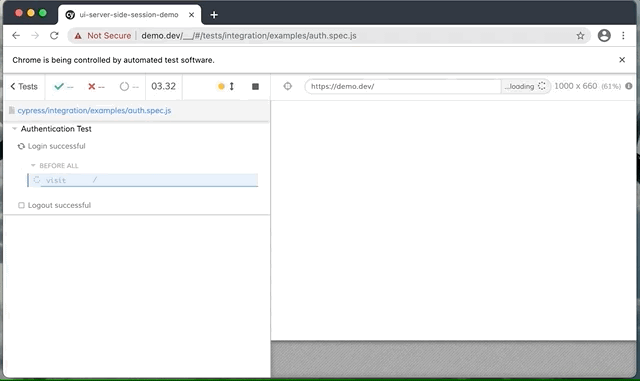
Author
Gladius
Website: thepro.io
Github: @gladius